The selective use of well-designed animations has been shown to improve user experience, and although the web is slowly transitioning towards HTML5 for web animations, its use also gives rise to some complications.
Scalable vector graphics (or SVGs) provide an accessible alternative, with a great API along with many other benefits, and they can be animated with a little creative use of Cascading Style Sheets.
Over the course of this article, we’re going to delve into all the other benefits of using SVGs and teach you exactly how to use them in combination with CSS to create animated logos.
Why Scalable Vector Graphics Are So Cool
Despite what the name implies, Scalable Vector Graphics is not a type of image file format; it’s an XML markup language (much like XHTML or XOXO). This specific markup language is used for creating vector-based two-dimensional images, which can be scaled up or down without any loss in resolution.
To put this into perspective, let’s take a look at the code behind a simple SVG file before moving on to talk about more of this format’s properties.
The example below reproduces a copy of Adobe’s logo by tracing a vector path and using the fill attribute to colorize it. Feel free to copy it using your preferred text editor (saving it as a .svg file) and then open it within your web browser to see the final result (note how the logo scales seamlessly as we discussed earlier).
<svg viewBox='0 0 105 93' xmlns='http://www.w3.org/2000/svg'></pre> <path d='M66,0h39v93zM38,0h-38v93zM52,35l25,58h-16l-8-18h-18z' fill='#ED1C24'/> </svg>
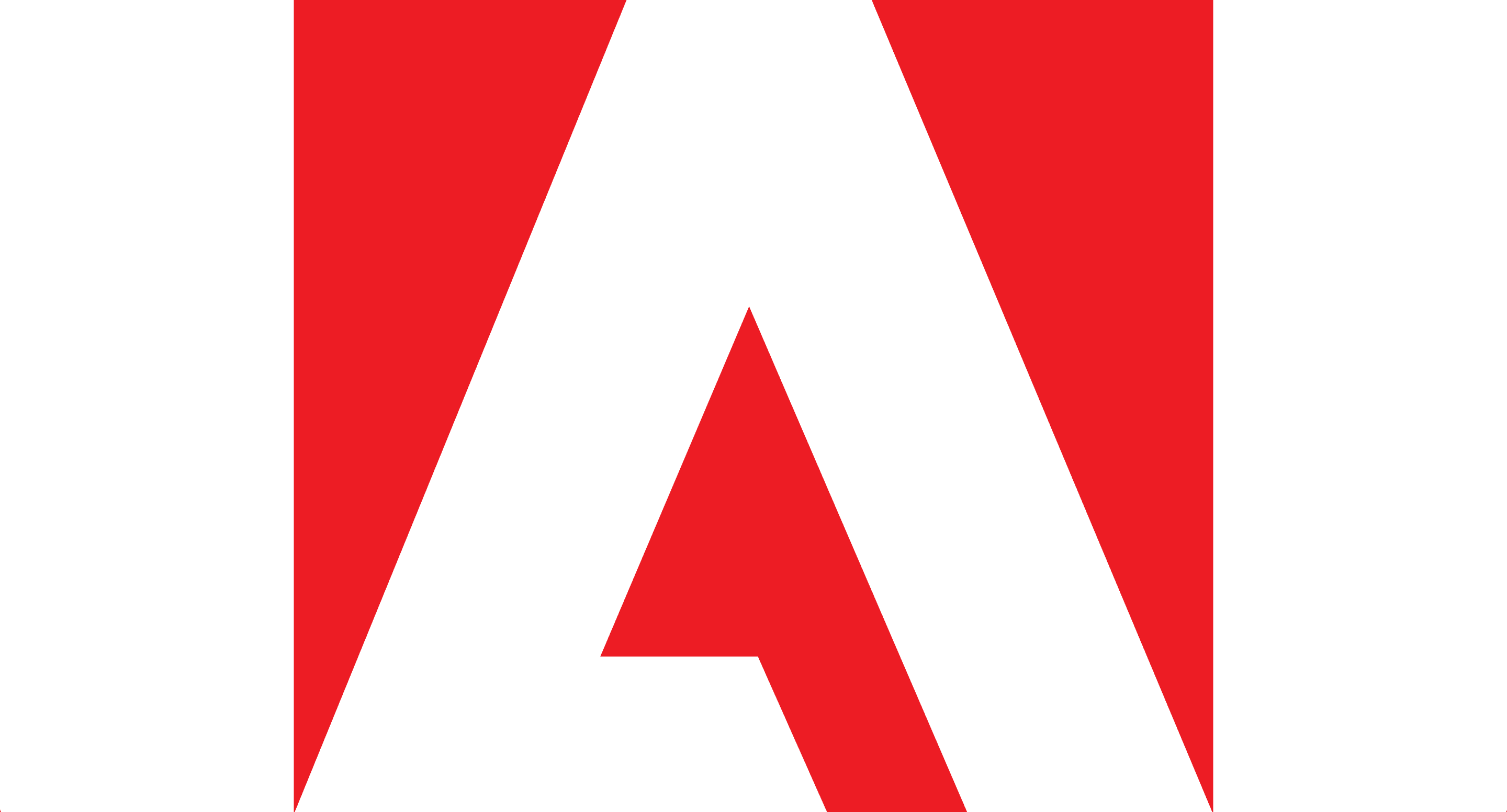
SVG in action.
As you can imagine, this can come in quite handy when building a responsive website. However, since the graphics are rendered using vectors, they tend to take up more data than necessary when it comes to complex images. Due to this fact, SVGs aren’t used often outside of graphics such as logos, infographics or regular graphs (although it does happen).
On top of being responsive, scalable vector graphics also have some built-in graphic effects (such as filters or blend modes) and have a very accessible DOM API. In fact, if you’re already familiar with CSS and JavaScript (which of course you are, you rockstar), you should be able to manipulate SVG files without too much hassle. This flexibility enables you to, for example, create SVG files server-side, and modify them easily without having to resort to using dedicated tools (which we will cover later on).
Lastly, SVGs can be easily animated using CSS, which you may have surmised from the title of this article (or the repeated references we’ve made to this fact), and we’ll get to that in a bit. But first, let’s go through the actual process of manipulating SVGs using dedicated tools.
How to Work With SVGs
As we mentioned earlier, you can dive into SVGs with your own two hands if that’s to your liking. Although it is a completely valid method, there are great tools available for directly creating and manipulating SVGs, which makes it inefficient to wrestle with the code for anything other than simple CSS styling or animations.
Adobe Illustrator, Inkscape and Sketch for Mac are the most popular options. In Illustrator’s case, the creation process is as simple as getting your image ready and then exporting it using the ‘Save as’ SVG option. When doing this, you might notice an option named SVG Profiles with several choices listed on a dropdown menu, like SVG Tiny (better suited for smartphones) and SVG Basic (an outdated profile meant to be used for PDAs), but we’re going to be sticking with SVG 1.1.
An unfortunate byproduct of using tools such as these to create SVGs files is that they can add a lot of metadata to the underlying code, which results in files bigger than they need to be. Naturally, this is a problem in web design, since you don’t want load times to increase due to poor optimization on your end. Scalable Vector Graphics must then be optimized (in most cases) before you can incorporate them into your website.
Let’s take a look at an example of an SVG file depicting the Android logo before and after optimization to illustrate this point:
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 96 105"></pre> <g fill="#97C024" stroke="#97C024" stroke-linejoin="round" stroke-linecap="round"> <path d="M14,40v24M81,40v24M38,68v24M57,68v24M28,42v31h39v-31z" stroke-width="12"/> <path d="M32,5l5,10M64,5l-6,10 " stroke-width="2"/> </g> <path d="M22,35h51v10h-51zM22,33c0-31,51-31,51,0" fill="#97C024"/> <g fill="#FFF"> <circle cx="36" cy="22" r="2"/> <circle cx="59" cy="22" r="2"/> </g> </svg>
Now we will work some optimization magic by removing spaces, default attributes, styles, and other non-essential data and we get:
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 96 105"><g style="fill:#97C024;stroke-linejoin:round;stroke:#97C024"><path d="M14 40v24M81 40v24M38 68v24M57 68v24M28 42v31h39v-31z" stroke-width="10"/><path d="M32 5l5 10M64 5l-6 10" stroke-width="2"/></g><path d="M22 35h51v10h-51zM22 33c0-31 51-31 51 0" fill="#97C024"/><g fill="#FFF"><circle cx="36" cy="22" r="2"/><circle cx="59" cy="22" r="2"/></g></svg>
Although the original SVG file was quite compact to begin with (0.5kB), the optimization process was able to reduce its filesize by 12.5% (to 0.4kB). On more complex images, you’ll notice even more extreme reductions in filesize and improvements in loading times when the SVGs are implemented into your website.
For simplicity’s sake, we recommend using tools such as the online SVG Optimizer created by Peter Collingridge or SVGO, a Node.js-based open-source app.
Now that we’ve learned what makes SVGs so useful and how to actually work with them, let’s see how you can use CSS to animate graphics created using this markup language.
Creating an Animated Logo With SVG and CSS
As we mentioned while talking about what makes SVGs so cool, they come with some built-in graphic effects which include animation capabilities. In fact, SVG has a native syntax solely for animations, referred to as SMIL (Synchronized Multimedia Integration Language), which enables you to animate the attributes of your graphics directly within the XML markup.
However, Internet Explorer never introduced support for SMIL and Chrome developers have talked about removing support for this syntax in favor of CSS and web animations. Using CSS to animate SVGs also has the advantage of being pretty simple in comparison with SMIL or JavaScript (although there are some libraries out there, such as Snap or Velocity, which aim to simplify this process).
Now, while scalable vector graphics can be integrated into websites either as embedded images, CSS backgrounds or inline graphics, you can only use CSS animations for the last option. This means we can only animate SVG markup which is directly within our HTML code.
Let’s take a look at some examples. Remember the SVG Adobe logo we went over earlier? We’re going to use a simple hover animation and transition effect to change its color. We begin by specifying a class for the vector path (which we will helpfully name adobe-logo), then adding the effects to that class in our style file. Here’s what the HTML would look like:
<!DOCTYPE html></pre> <html> <head> <title>SVG Transitions</title> <link rel="stylesheet" href="css/style.css"> </head> <body> <!-- The Adobe Logo recreated using SVG --> <svg viewBox='0 0 105 93' xmlns='http://www.w3.org/2000/svg'> <path class="adobe-logo" d='M66,0h39v93zM38,0h-38v93zM52,35l25,58h-16l-8-18h-18z' fill='#ED1C24'/> </svg> </body> </html>
Now we move onto our styles.css file:
/* -------------------------- Here we add a little padding and specify a width. --------------------------- */ body { padding-top: 5em; } svg { width: 33.32%; float: left; } /* -------------------------- Now we add the actual animation. --------------------------- */ .adobe-logo { transition: .3s ease-out; } .adobe-logo:hover { fill: #3881cc; }
Which would look like this:
If instead of a hover animation, you want to add a fill and strokes effect to the same SVG graphic, the code would be something like:
/* -------------------------- Fill and strokes. --------------------------- */ .adobe-logo { stroke: #fee16e; } .adobe-logo:hover { fill: #feae6e; stroke-width: 8; } .adobe-logo { transition: .3s ease-out; }
When it’s all set, here’s what that would look like:
These are only a couple of simple examples of what you can accomplish using CSS when it comes to animating SVG graphics, but if you want some real inspiration, let’s take a look at an example of what can be accomplished using other techniques such as groupings, transformations, rotating, scaling, and even CSS keyframes:
Pretty neat, right? As you can see, CSS can be used to achieve some pretty complex stuff in combination with SVGs. If you don’t want to comb through CodePen and CSSDeck to find more cool examples, take a look at 30 Awesome SVG Animation For Your Inspiration.
Finally, it should be mentioned that CSS animation does have a few limitations. For example, it won’t allow you to change the actual shape of an element; for that you’ll need to resort to JavaScript animations. For further reading on this topic, take a look at 8 JavaScript Libraries to Animate SVG.
Conclusion
If used properly, animations can elevate the user experience and overall design of a site. Although SVGs in combination with CSS provide an elegant way to implement these, there’s plenty of other methods you can also try until you find the one that best satisfies your needs.
If you do choose to go with CSS animations for SVGs, remember to follow the advice above:
- Pick a compatible graphic editor tool.
- Remember to always optimize your SVG code.
- Keep in mind that you can only implement ‘simple’ animations using this technique.
Check out the additional resources we listed above if you want to take a look at some of the awesome effects designers have achieved using CSS in combination with scalable vector graphics. Who knows? You might find some inspiration there.
What’s your preferred animation method for your designs? Let us know in the comments section below!
Article thumbnail image by Giingerann / shutterstock.com
Amazing !!!
Thanks for the Post
Do this SVG perform good on GTMatrix Check?
Hello! I’m not too sure what you mean? Could you rephrase your question please?
I first heard about using SVG files from Seanwes. Definitely something I’m interested in utilizing in the future.
Glad the article has inspired you, Monica!
Thank you for Nice post…
This is awesome and thank you for posting this! It makes me think about where we can potentially take logos, a company’s design, branding, and marketing message, and then implement the design on a website. Time will tell!
Thanks Tom!
Excellent! Thank you!
Does anyone know the name of the theme used in this blog
We use a custom theme.
Very cool! =D
Excellent Info I never though that some code are work like this i just start learning css and JavaScript and after watching your post i feel amazing thank for sharing this Hope to see some more good stuffs from you in future.
oh wow. I didn’t know how you can do this by manipulating svg’s but that’s cool. I like to try it out sometime.
That’s great, but stock WordPress refuses to support svg files. They claim the format is vulnerable to some xss exploit or something. Which is an absolute shame, the platform powering over 25% of the web refuses to allow the use of the perfect image format for the web. It is ridiculous. Something needs to be done about it. Soon.
Just add this to your WordPress themes function file:
function cc_mime_types($mimes) {
$mimes[‘svg’] = ‘image/svg+xml’;
return $mimes;
}
add_filter(‘upload_mimes’, ‘cc_mime_types’);
Hey presto, WordPress supports SVG’s – no need for a plugin for something so simple!
To also allow SVG files upload to the Theme Customizer you need to add the following snippet too:
via: http://wordpress.stackexchange.com/a/159615/2015
$wp_customize->add_control(
new WP_Customize_Image_Control(
$wp_customize,
‘themeslug_logo’,
array(
‘label’ => __( ‘Logo’, ‘themeslug’ ),
‘section’ => ‘themeslug_logo_section’,
‘settings’ => ‘themeslug_logo’,
‘extensions’ => array( ‘jpg’, ‘jpeg’, ‘gif’, ‘png’, ‘svg’ ),
)
)
);
Thanks Kevin and Piet for the alternative approach!
As of now, a free plugin like this is probably the best bet: https://wordpress.org/plugins/svg-support/
Well Nathan I think that plugin needs to be apart of the article. At least to talk about WordPress and SVG.
Apart? Think you mean “a part”.
You’re welcome.
Great – thanks for the article. You mention you can’t animate the shape of an element with CSS, however I suggest looking at this link. shape-inside: polygons can do that – It’s not great yet, but certainly doable – http://codepen.io/SaraSoueidan/pen/17dd591f451f4757366faf3c9246504b
Great post. Your post explained how to create an animated logo with SVG and CSS very well. Thanks.
Excellent post!
Thanks!
Very good info, will have to save this for later use 🙂
Nice…. I would love to try svg on my site now.
Right?! It’s so cool!
nice post! …get rid of flash folks!
Did you see that flash has now been replaced by Adobe with Adobe Animate CC?
http://www.elegantthemes.com/blog/resources/an-overview-of-adobe-cc-apps-web-designers-should-be-using
Nice post Tom! (thumbs up)